Unity C# 音ゲーの作り方講座の第4回目、「ノーツをスクリプトから生成して配置しよう」をチュートリアル形式で説明していきます。
前回は「TextMeshProや画像を配置してゲーム結果を表示させよう」でゲーム画面の設計を行いました。
ここでは、第1回の講座で作成したmusicboxゲームオブジェクトをスクリプトから生成していきます。このことをインスタンスの生成と呼びます。
Unityでゲーム制作する際にゲームオブジェクトをスクリプトから生成したくなることはよくあります。スクリプト慣れも兼ねてここで実際にやってみましょう。
音ゲーのmusicboxオブジェクトのプレハブを作成する
オブジェクトのインスタンスを生成する前にプレハブにします。
プレハブとは、複数のオブジェクトを1つのアセットとしてまとめてくれる機能です。プレハブを一度作っておけばそのオブジェクトを何度でも再利用できます。
プレハブの作り方を説明していきます。
まず、『Assets』フォルダーの中に『Resources』フォルダーを作成してください。
ヒエラルキーの中にある『musicbox.pink』オブジェクトを選択して『Resources』フォルダーにドロップさせてください。
これで1つ目のプレハブ作成は完了です。
同様に残り4つの『musicbox』オブジェクトを『Resources』フォルダーにドロップさせてください。
以下のようになっていればプレハブの作成は完了です。
プレハブにしたmusicboxのインスタンスを生成させる
musicboxをプレハブにしたのでインスタンスを生成していきましょう。
インスタンスの生成を行うスクリプトを作成して配置したい場所をC#スクリプトで入力して作成していきます。
『Resources』フォルダーの中でスクリプトを作成して名前を『Resourcespink』に変更してください。
フォルダ内にResourcespink.csが作られます。
初めにサンプルスクリプトを以下に掲載します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 |
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Resourcespink : MonoBehaviour { // Start is called before the first frame update void Start() { //プレハブをGameobject型で取得 GameObject objpink = (GameObject)Resources.Load("musicbox.pink"); //プレハブを元にインスタンスを生成 Instantiate(objpink, new Vector3(-3.02f, 0.35f, 25.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 30.5f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 39.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 42.5f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 49.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 60.5f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 68.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 71.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 86.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 90.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 100.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 111.5f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 118.5f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 126.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 128.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 142.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 145.5f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 148.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 152.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 161.5f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 180.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 187.5f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 193.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 196.5f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 219.5f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 225.5f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 260.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 265.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 270.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 227.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 280.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 289.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 294.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 298.5f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 307.5f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 313.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 316.5f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 322.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 326.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 331.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 336.5f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 338.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 339.5f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 343.5f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 350.5f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 356.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 361.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 365.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 370.0f), Quaternion.identity); Instantiate(objpink, new Vector3(-3.02f, 0.35f, 375.0f), Quaternion.identity); } } |
『Resourcespink』スクリプトの解説を行います。
以下のコードはUnityの中から『musicbox.pink』オブジェクトのプレハブを探すという内容です。
1 2 |
//プレハブをGameobject型で取得 GameObject objpink = (GameObject)Resources.Load("musicbox.pink"); |
次のコードは、探したプレハブを元にインスタンスを生成するコードです。
1 2 |
//プレハブを元にインスタンスを生成 Instantiate(objpink, new Vector3(-3.02f, 0.35f, 25.0f), Quaternion.identity); |
Vecter3の横にある( )の中に配置したいXYZの順で数値を入力しています。
『musicbox』オブジェクトはZ方向に流れるためオブジェクトの色ごとにXYの数値は固定してZの数値のみ変更しています。
スクリプトの作成が完了したらヒエラルキーの中で右クリックして『空のオブジェクトを作成』を選択して名前を『Instancepink』に変更してください。
『Instancepink』は、musicboxオブジェクトの色を把握できるように色の名前をつけています。
『Instancepink』オブジェクトのインスペクターに先ほど作成した『Resourcespink』スクリプトをドロップさせてください。
『musicbox.pink』オブジェクトのインスタンス生成が出来ているか確認するためにゲームの実行ボタンを押してみましょう。
ゲーム画面の上にある実行ボタン▶︎を押してください。
以下のようにロードの奥の方で『musicbox.pink』オブジェクトが増えていたらインスタンスの生成は完了です(見えづらかったらScene画面も見てくださいたくさんのオブジェクトが生成されていることが確認できます)。
残りの4つの音ブロックのインスタンスを生成させていきましょう。
以下にスクリプト名と完成版コード、Instanceオブジェクトの名前を記載します。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Resourcesgreen : MonoBehaviour { // Start is called before the first frame update void Start() { //プレハブをGameobject型で取得 GameObject objgreen = (GameObject)Resources.Load("musicbox.green"); //プレハブを元にインスタンスを生成 Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 29.0f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 44.0f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 58.5f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 74.5f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 90.5f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 94.0f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 100.0f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 115.0f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 129.0f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 144.0f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 158.5f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 174.5f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 190.5f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 194.0f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 200.0f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 216.0f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 229.0f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 235.0f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 250.0f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 280.0f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 300.0f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 302.0f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 315.0f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 315.0f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 333.0f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 340.5f), Quaternion.identity); Instantiate(objgreen, new Vector3(-0.99f, 0.35f, 371.0f), Quaternion.identity); } } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 |
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Resourcesblue : MonoBehaviour { // Start is called before the first frame update void Start() { //プレハブをGameobject型で取得 GameObject objblue = (GameObject)Resources.Load("musicbox.blue"); //プレハブを元にインスタンスを生成 Instantiate(objblue, new Vector3(1.01f, 0.35f, 25.0f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 27.0f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 57.0f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 58.5f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 74.5f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 77.0f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 94.0f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 106.0f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 110.5f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 119.0f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 120.5f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 126.0f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 157.0f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 158.5f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 174.5f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 178.0f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 179.0f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 194.0f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 205.0f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 221.0f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 234.5f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 260.5f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 280.5f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 305.5f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 312.0f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 331.5f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 339.0f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 347.5f), Quaternion.identity); Instantiate(objblue, new Vector3(1.01f, 0.35f, 369.0f), Quaternion.identity); } } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Resourcesorange : MonoBehaviour { // Start is called before the first frame update void Start() { //プレハブをGameobject型で取得 GameObject objorange = (GameObject)Resources.Load("musicbox.orange"); //プレハブを元にインスタンスを生成 Instantiate(objorange, new Vector3(3.05f, 0.35f, 27.0f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 34.5f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 47.0f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 55.5f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 65.5f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 70.5f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 87.0f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 108.0f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 113.5f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 124.0f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 134.5f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 147.0f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 155.0f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 165.0f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 170.5f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 177.5f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 187.5f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 196.5f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 203.0f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 211.0f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 220.0f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 231.5f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 244.0f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 258.0f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 266.5f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 279.0f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 282.5f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 286.0f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 294.0f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 308.0f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 313.5f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 324.0f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 334.5f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 347.0f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 355.0f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 365.0f), Quaternion.identity); Instantiate(objorange, new Vector3(3.05f, 0.35f, 370.5f), Quaternion.identity); } } |
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
using System.Collections; using System.Collections.Generic; using UnityEngine; public class Resourcesbakudan : MonoBehaviour { // Start is called before the first frame update void Start() { //プレハブをGameobject型で取得 GameObject objbakudan = (GameObject)Resources.Load("musicbox.bakudan"); //プレハブを元にインスタンスを生成 //road.pinkに配置する Instantiate(objbakudan, new Vector3(-3.02f, 0.35f, 109.0f), Quaternion.identity); Instantiate(objbakudan, new Vector3(-3.02f, 0.35f, 163.0f), Quaternion.identity); Instantiate(objbakudan, new Vector3(-3.02f, 0.35f, 174.0f), Quaternion.identity); Instantiate(objbakudan, new Vector3(-3.02f, 0.35f, 183.0f), Quaternion.identity); Instantiate(objbakudan, new Vector3(-3.02f, 0.35f, 209.0f), Quaternion.identity); Instantiate(objbakudan, new Vector3(-3.02f, 0.35f, 263.0f), Quaternion.identity); Instantiate(objbakudan, new Vector3(-3.02f, 0.35f, 274.0f), Quaternion.identity); Instantiate(objbakudan, new Vector3(-3.02f, 0.35f, 283.0f), Quaternion.identity); //road.greenに配置する Instantiate(objbakudan, new Vector3(-0.99f, 0.35f, 305.0f), Quaternion.identity); Instantiate(objbakudan, new Vector3(-0.99f, 0.35f, 312.0f), Quaternion.identity); Instantiate(objbakudan, new Vector3(-0.99f, 0.35f, 331.5f), Quaternion.identity); Instantiate(objbakudan, new Vector3(-0.99f, 0.35f, 339.0f), Quaternion.identity); Instantiate(objbakudan, new Vector3(-0.99f, 0.35f, 347.5f), Quaternion.identity); Instantiate(objbakudan, new Vector3(-0.99f, 0.35f, 369.0f), Quaternion.identity); //road.blueに配置する Instantiate(objbakudan, new Vector3(1.01f, 0.35f, 124.5f), Quaternion.identity); Instantiate(objbakudan, new Vector3(1.01f, 0.35f, 144.5f), Quaternion.identity); Instantiate(objbakudan, new Vector3(1.01f, 0.35f, 154.5f), Quaternion.identity); Instantiate(objbakudan, new Vector3(1.01f, 0.35f, 176.5f), Quaternion.identity); Instantiate(objbakudan, new Vector3(1.01f, 0.35f, 184.5f), Quaternion.identity); Instantiate(objbakudan, new Vector3(1.01f, 0.35f, 224.5f), Quaternion.identity); Instantiate(objbakudan, new Vector3(1.01f, 0.35f, 244.5f), Quaternion.identity); Instantiate(objbakudan, new Vector3(1.01f, 0.35f, 254.5f), Quaternion.identity); Instantiate(objbakudan, new Vector3(1.01f, 0.35f, 276.5f), Quaternion.identity); //road.orangeに配置する Instantiate(objbakudan, new Vector3(3.05f, 0.35f, 117.0f), Quaternion.identity); Instantiate(objbakudan, new Vector3(3.05f, 0.35f, 122.0f), Quaternion.identity); Instantiate(objbakudan, new Vector3(3.05f, 0.35f, 126.0f), Quaternion.identity); Instantiate(objbakudan, new Vector3(3.05f, 0.35f, 141.0f), Quaternion.identity); Instantiate(objbakudan, new Vector3(3.05f, 0.35f, 150.0f), Quaternion.identity); Instantiate(objbakudan, new Vector3(3.05f, 0.35f, 163.5f), Quaternion.identity); Instantiate(objbakudan, new Vector3(3.05f, 0.35f, 189.0f), Quaternion.identity); } } |
5つの設定完了後、ゲームの再生ボタンを押してGameやScene画面が以下のようになっていればインスタンスの生成は完了です。
スクリプトで音を生成するorプレハブを直接画面に配置して設定する どっちのやり方がいい?
今回は作っておいたプレハブをスクリプトから読み込ませて生成する方法を学びました。
が、音ゲーを作るという点ではスクリプトの数値をいじるよりUnityエディタ上でノーツとなる音ブロックを動かしながら視覚的に配置・移動させて微調整する方が便利かもしれませんよね。
スクリプトで書いて変更していちいちUnityエディタで読み込ませてると時間もかかりますし・・・
いずれにせよまじめに音ゲーを仕上げていく場合は音の配置の微調整は必要になります。
外部のツールやデータを読み込ませて使いたい場合はスクリプトからノーツを生成する方がいいでしょうし、Unityだけで音ゲーを作る場合は視覚的に動かせるようにプレハブを一つ一つ手で置いていくほうがいいかもしれません。
そのあたりは自分の開発スタイルに合わせて最適な方法を選んで作っていけばよいでしょう。
音ゲーの作り方 第4回のまとめと次回
第4回目の開発お疲れさまでした。
今回は、オブジェクトのプレハブを作成してスクリプトから生成させる方法・スクリプトから生成するのが本当に良いのかどうかを解説しました。
インスタンスの生成はゲーム進行と共にスクリプトでゲームオブジェクトを生成したい時に利用されます。
この機能を用いて今回は音ゲーに必須となるノーツの生成・配置を実現しました。
しかし、音ゲーのようにオブジェクトの位置の微調整をスピーディーに行いたいならUnityエディタ上で直接配置をチューニングするのも良いでしょう。
次回は、第5回の「Unity C# 音ゲーの作り方5 musicboxをロードの上で流れるようにしてボタンの仕組みを作成しよう」です。
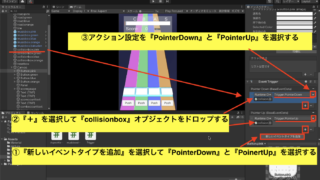
コメント